In the last blog, I discussed about how to programmatically add controls at runtime. This blog, we'll do just the opposite of that - removing controls programmatically.
The Controls collection offers a few methods that we can use to remove controls at runtime (Clear, Remove, RemoveAt).
Be careful when you call Remove or RemoveAt method though, as the Count property as well as the collection will be updated instantaneously and might produce undesirable result. Consider the following code snippet:
Dim i as Integer
With Me.Controls
For i = 0 to .Count - 1
If TypeOf .Item(i) is CheckBox Then
.RemoveAt(i)
Next
End With
This will work just fine if you have only one checkbox. However, if you have more than one checkboxes on the form, then it will remove every other one as the control collection is updated as soon as a control is removed resulting in a change in the Count property which messes things up and eventually throw an index out of range error.
To work around this, we'll have to remove the controls from the high end of the Count property as follow:
Dim i as Integer
With Me.Controls
For i = .Count - 1 To 0 Step -1
If TypeOf .Item(i) is CheckBox Then
.RemoveAt(i)
Next
End With
You can download the code for this blog here.
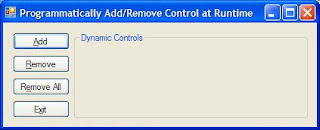
The Controls collection offers a few methods that we can use to remove controls at runtime (Clear, Remove, RemoveAt).
Be careful when you call Remove or RemoveAt method though, as the Count property as well as the collection will be updated instantaneously and might produce undesirable result. Consider the following code snippet:
Dim i as Integer
With Me.Controls
For i = 0 to .Count - 1
If TypeOf .Item(i) is CheckBox Then
.RemoveAt(i)
Next
End With
This will work just fine if you have only one checkbox. However, if you have more than one checkboxes on the form, then it will remove every other one as the control collection is updated as soon as a control is removed resulting in a change in the Count property which messes things up and eventually throw an index out of range error.
To work around this, we'll have to remove the controls from the high end of the Count property as follow:
Dim i as Integer
With Me.Controls
For i = .Count - 1 To 0 Step -1
If TypeOf .Item(i) is CheckBox Then
.RemoveAt(i)
Next
End With
You can download the code for this blog here.
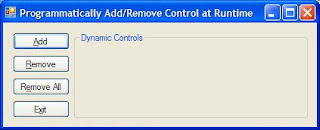
No comments:
Post a Comment